Similarly use updateOne, updateMany, deleteOne, deleteMany to perform Update or Delete operations
Number of calls to the above functions are limited to 100 from within a single execution of a Server Side Script or Cloud Function.
If you want to insert more than 100 rows in a single execution, always use db.insertMany(...) instead of multiple db.insertOne(...) calls.
Duplicating a Record
constdbRow=awaitdb.findOne("DataSourceName", { filter: [{ headerId: { eq:101 } }],});constnewRow=utils.toNewRow(dbRow); // this will remove all WHO columns and other internal keysdeletenewRow.headerId; // remove fields that are auto populated by the platformconstinsertedRow=awaitdb.insertOne("DataSourceName", newRow);Logger.log(JSON.stringify(insertedRow));
Throwing a Functional Error
rows.forEach((row) => {if (row.someField ==="invalid") {thrownewUserError(`Some Field is invalid ${row.someField}!`); }});
constresult=awaitdb.executeQuery( // returns Record<string, unknown>[]`SELECT * FROM SOME_TABLE WHERE SOME_COLUMN = ?`, [session.userName()] );constvalue=awaitdb.executeString( // returns string | undefined`SELECT 'Some string value'`, [] );awaitdb.executeUpdate(`UPDATE SOME_TABLE SET SOME_COLUMN = ? WHERE SOME_COLUMN = ?`, ['X','Y'] );
You can pass true (boolean) as the 3rd parameter to query against the cloudio schema instead of your application schema
Awaiting for all Promises to completion
Wrong Way ❌
rows.forEach(async (row) => {// make async calls for each rowawaitdb.someAsyncFn(...);});// this code will exit even before all the above promises are resolved
Right Way ✅
constcollectedResponse=awaitPromise.all(rows.map(async (row) => {// make async calls for each rowawaitdb.someAsyncFn(...);return"someThing";}));// this code will wait for all the promises to be resolved before exiting
Sending User Notification
constroleCode="myRole";constempId=123;constsubject='Hi there!';// notification body can be formatted with Markdown or basic HTML tagsconstbody=`#### h4##### h5###### h6A sample paragraph...Don't put tabs or spaces in front of your paragraphs.First line with two spaces after. And the next line.First line with the HTML tag after.<br>And the next line.> QuoteSome **bold** *italic* ***bold italic*** text...Alerts: alert_warning, alert_info, alert_success, alert_error\`\`\`alert_warningA warning alert...!\`\`\`Unordered list- one- two- threeOrdered list1. one1. two1. threeInternal link [click here](<#/howto/edit-employee?empId=${empId}&role=${roleCode}&some=new %thing>) to navigate to the employee edit page within the current tab.
Enclose the URL within angular braces <...> to URL encode space, % etc.External link [cloudio.io](https://cloudio.io). Opens in a new tab.Render Image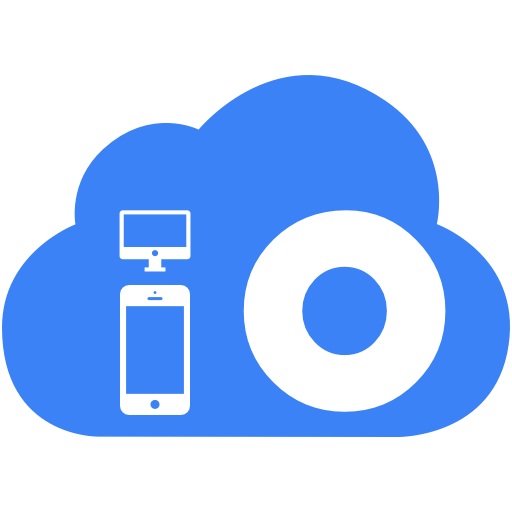Use HTML<a href="https://www.example.com/my great page">link</a><img src="https://dev.cloudio.io/images/io_icon.png"/>`;// send the notification// to a user e.g. adminawaitdb.notifyUser('admin', subject, body);// to a set of users e.g. admin, username2awaitdb.notifyUser(['admin','username2'], subject, body);// to all users assigned to a roleawaitdb.notifyRole('my-app','developer', subject, body);// to all users assigned to a set of rolesawaitdb.notifyRole('my-app', ['administrator','developer'], subject, body);
Sending Email
// Text Emailconstemail= { to:'example@domain.com', subject:'Hi there!', text:'email body', };awaitdb.sendEmail(email);// HTML EmailconsthtmlBody=awaitdb.processMustacheTemplate(templateString, dataObject):constemail= { to:'example@domain.com', subject:'Hi there!', text:'HTML Only Email', html: htmlBody };awaitdb.sendEmail(email);
Triggering Workflow
constwfUid="..."; // Workflow UID that can be optained from Edit Workflow Panelconstversion=1; // Workflow Version from Edit Workflow Panelconststate= {}; // Any JSON object to set the initial state of the Workflowawaitdb.startWorkflow(wfUid, version,"Some description", state);